| Tiago Coimbra
5. Remote Code Execution (RCE)
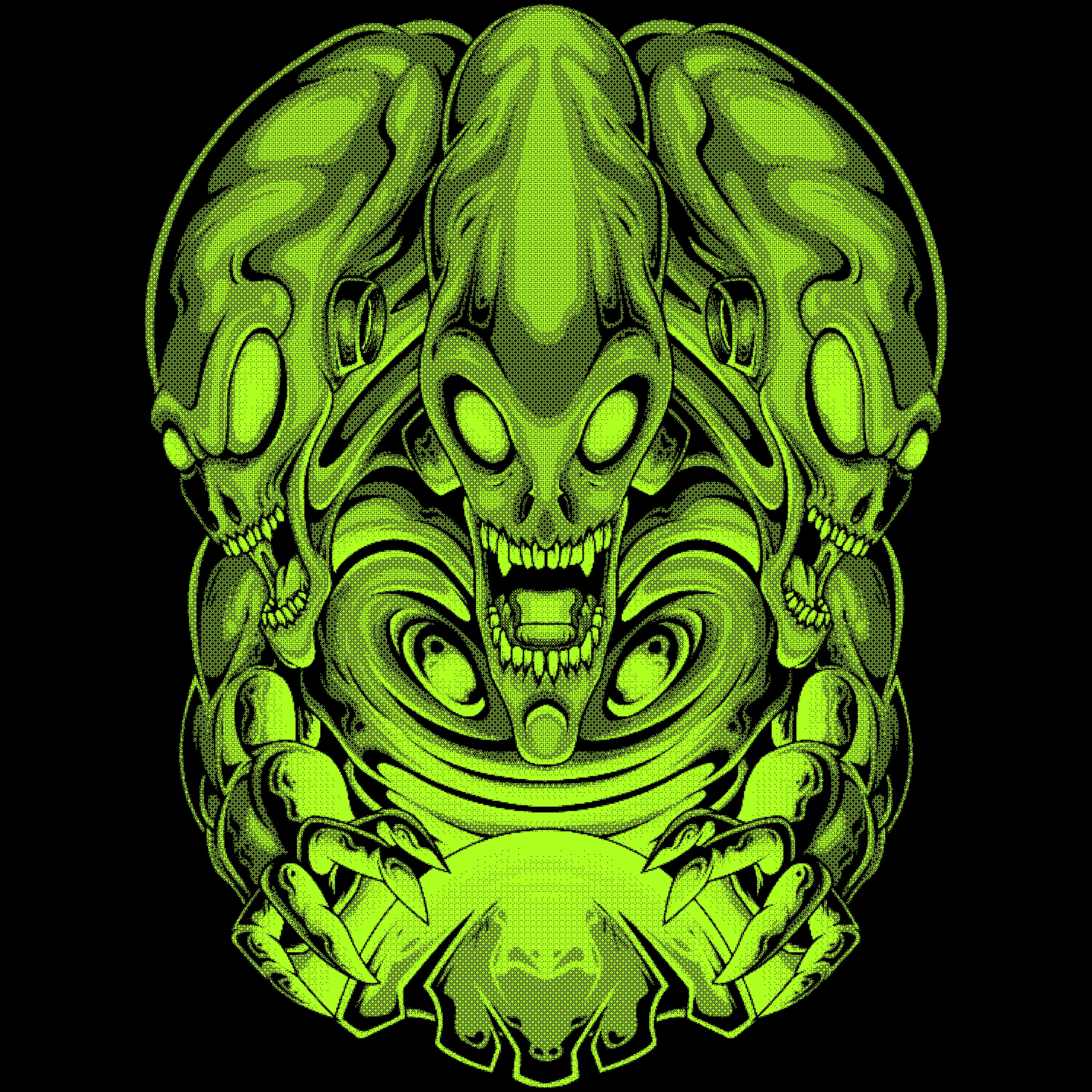
5. Remote Code Execution (RCE)
Category: #Code-Execution-Attack
Attack:
RCE allows attackers to execute arbitrary code on a server by exploiting vulnerabilities in the code or environment.
Attack Code Example (RCE via User Input):
GET /run?cmd=rm+-rf+/
This attack injects a malicious command (rm -rf /
) through a vulnerable web application that allows user input to be passed directly to the shell.
Vulnerable Code (Python using subprocess
):
import subprocess
@app.route('/run', methods=['GET'])
def run_command():
cmd = request.args.get('cmd')
subprocess.run(cmd, shell=True) # Dangerous! Allows RCE
Remediation Steps:
- Input Validation: Validate all user inputs and reject any unexpected or malicious values.
- Avoid Shell Commands: Avoid using shell commands for user inputs. Use parameterized functions or libraries.
- Escape User Inputs: If shell execution is necessary, ensure all inputs are properly escaped.
Safe Code (Using parameterized commands in Python):
import subprocess
@app.route('/run', methods=['GET'])
def run_command():
allowed_commands = ['ls', 'cat', 'echo']
cmd = request.args.get('cmd').split()[0]
if cmd not in allowed_commands:
abort(403) # Reject dangerous commands
subprocess.run([cmd], check=True) # Safe execution without shell=True
Reference:
OWASP Command Injection