| Tiago Coimbra
10. Password Attacks (Brute Force, Credential Stuffing)
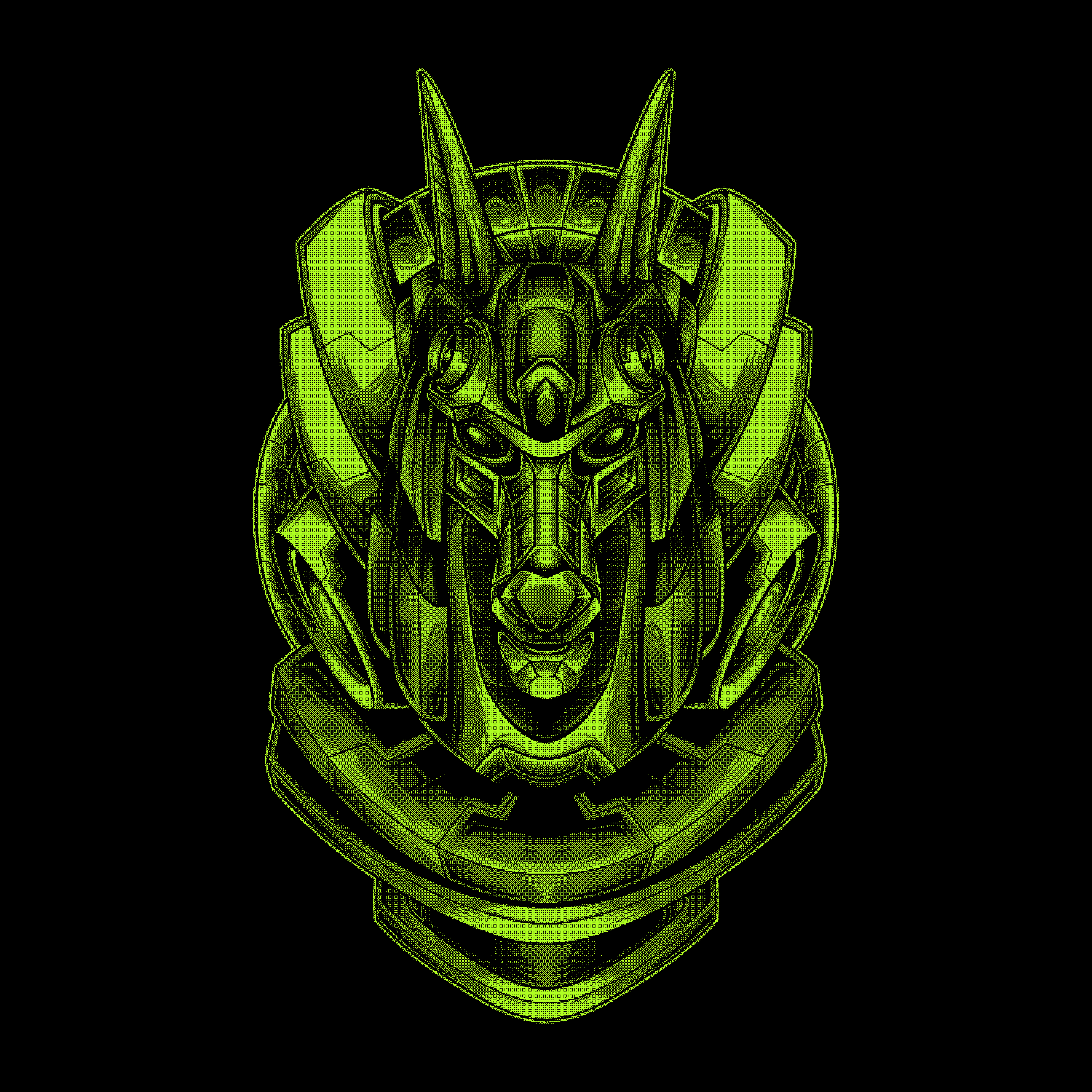
Password Attacks (Brute Force, Credential Stuffing)
Category: #Authentication-and-Session-Attack
Attack:
Password attacks include brute force attacks, where attackers try multiple password combinations, and credential stuffing, where attackers use leaked credentials to log in.
Attack Code Example (Brute Force):
POST /login # Automated tool tries millions of password combinations to find the correct one
Vulnerable Code (No account lockout or rate limiting):
@app.route('/login', methods=['POST'])
def login():
username = request.form['username']
password = request.form['password']
user = authenticate(username, password)
return "Login successful" if user else "Login failed"
Remediation Steps:
- Account Lockout Mechanism: Lock accounts after a set number of failed login attempts.
- Rate Limiting: Implement rate limiting to prevent multiple rapid login attempts.
- Multi-Factor Authentication (MFA): Use MFA to add an additional layer of security beyond the password.
- Use Secure Hashing Algorithms: Store passwords securely using strong hash functions like bcrypt or Argon2.
Safe Code (Account lockout after multiple failed login attempts):
@app.route('/login', methods=['POST'])
def login():
username = request.form['username']
password = request.form['password']
if session.get('failed_attempts', 0) >= 5:
abort(403) # Lock account after 5 failed attempts
user = authenticate(username, password)
if not user:
session['failed_attempts'] = session.get('failed_attempts', 0) + 1
return "Login failed", 401
session['failed_attempts'] = 0 # Reset on successful login
return "Login successful"
In this code example, we enforce account lockout after 5 failed login attempts to mitigate brute force attacks. You can further enhance it by adding rate limiting to slow down repeated requests.
Remediation Steps Recap:
- Account Lockout: Lock accounts after a number of failed login attempts.
- Rate Limiting: Prevent rapid successive login attempts from the same IP.
- Use Multi-Factor Authentication (MFA): Add MFA to secure authentication.
- Strong Password Hashing: Store passwords using secure hashing algorithms like bcrypt.
Reference: OWASP Authentication Cheat Sheet