| Tiago Coimbra
7. Denial of Service (DoS) / Distributed Denial of Service (DDoS)
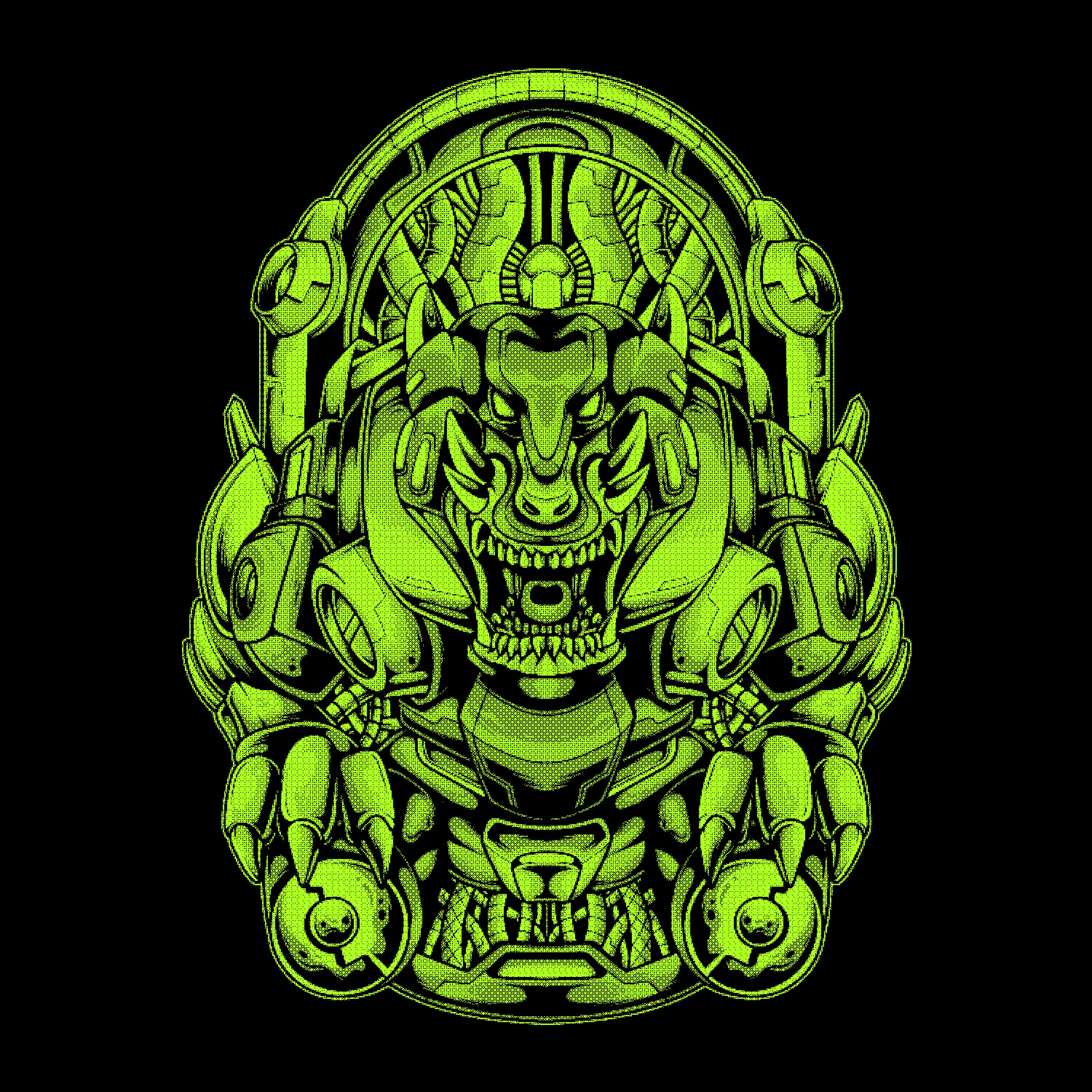
Denial of Service (DoS) / Distributed Denial of Service (DDoS)
Category: #Network-Based-Attack
Attack:
Denial of Service (DoS) and Distributed Denial of Service (DDoS) attacks aim to overload a service with traffic, rendering it unavailable to legitimate users.
Attack Code Example (DDoS Request Flooding):
POST /login # Flood the server with millions of login requests to exhaust resources
Attackers can flood the login endpoint with millions of requests, overwhelming the server and causing service disruption.
Vulnerable Code (No Rate Limiting):
@app.route('/login', methods=['POST'])
def login():
# Process login request without rate limiting or protections
username = request.form['username']
password = request.form['password']
user = authenticate(username, password)
return "Login successful" if user else "Login failed"
Remediation Steps:
- Rate Limiting: Implement rate limiting to restrict the number of requests a user or IP address can make in a certain time period.
- CAPTCHA: Use CAPTCHAs on sensitive forms to prevent bots from spamming requests.
- Web Application Firewall (WAF): Deploy a WAF to block malicious traffic patterns and protect against DDoS attacks.
- Content Delivery Networks (CDNs): Use CDNs with built-in DDoS protection to absorb large-scale attacks.
Safe Code (Rate limiting with Flask-Limiter):
from flask_limiter import Limiter
app = Flask(__name__)
limiter = Limiter(app, key_func=get_remote_address)
@app.route('/login', methods=['POST'])
@limiter.limit("5 per minute") # Restrict to 5 login attempts per minute
def login():
username = request.form['username']
password = request.form['password']
user = authenticate(username, password)
return "Login successful" if user else "Login failed"
Reference:
OWASP DoS Prevention Cheat Sheet