| Tiago Coimbra
4. Directory Traversal
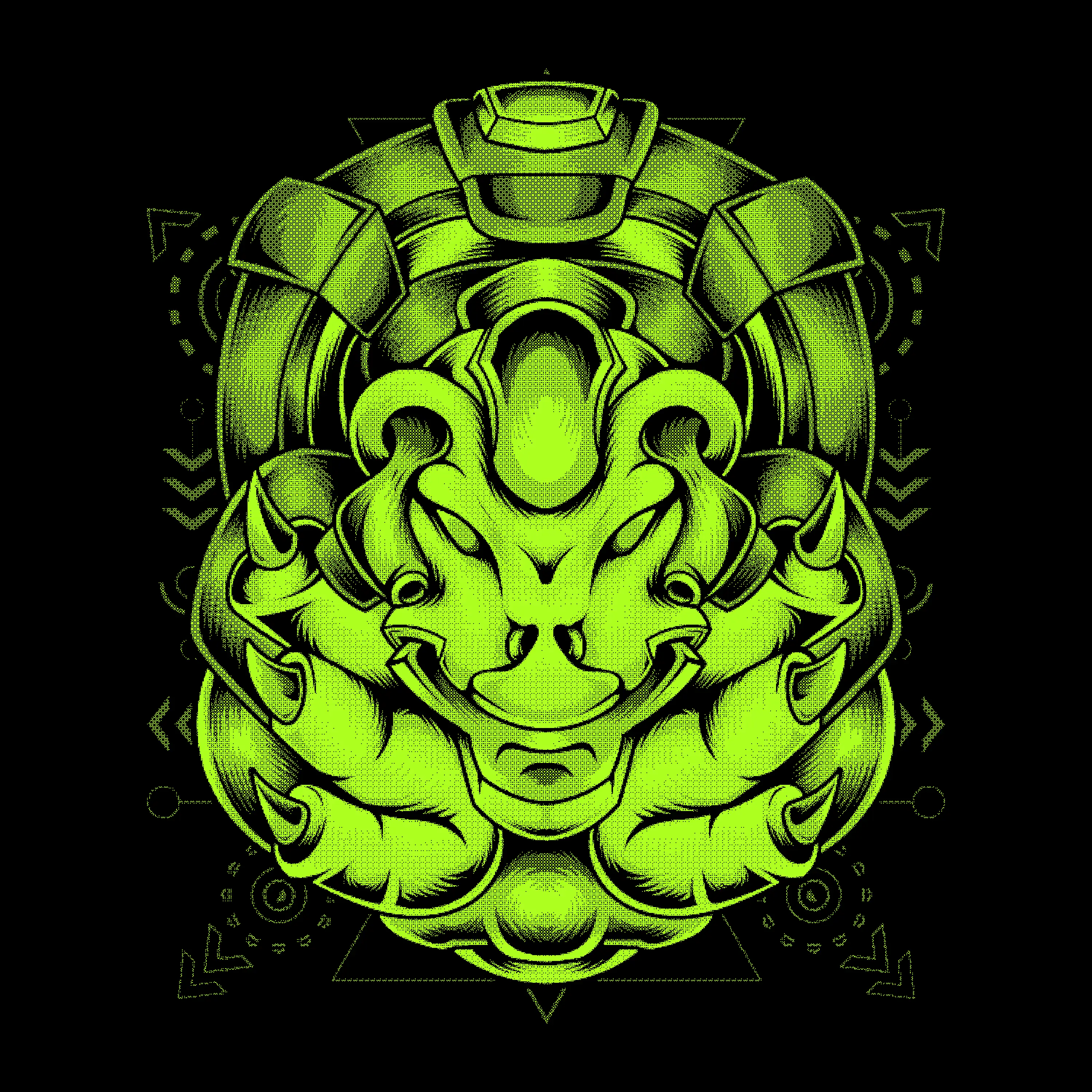
4. Directory Traversal
Category: #File-Based-Attack
Attack:
Directory Traversal allows attackers to access files and directories stored outside the web root by manipulating file paths.
Attack Code Example (Directory Traversal):
GET /download?file=../../etc/passwd
This request attempts to retrieve the /etc/passwd
file by traversing the directory structure.
Vulnerable Code (Python Flask):
@app.route('/download', methods=['GET'])
def download_file():
filename = request.args.get('file')
return send_file(filename) # No input validation, vulnerable to directory traversal
Remediation Steps:
- Canonicalization: Convert file paths to their canonical form before processing.
- Input Validation: Validate and sanitize all user inputs for file paths.
- Use Whitelisting: Limit file access to predefined directories.
Safe Code (Path Canonicalization in Python):
import os
def secure_file_path(filename):
base_dir = '/safe/directory/'
filename = os.path.normpath(filename) # Normalize path to avoid directory traversal
file_path = os.path.join(base_dir, filename)
if not file_path.startswith(base_dir):
abort(403) # Invalid file path
return file_path
@app.route('/download', methods=['GET'])
def download_file():
filename = request.args.get('file')
secure_filename = secure_file_path(filename)
return send_file(secure_filename)
Reference:
OWASP Path Traversal