| Tiago Coimbra
3. Cross-Site Request Forgery (CSRF)
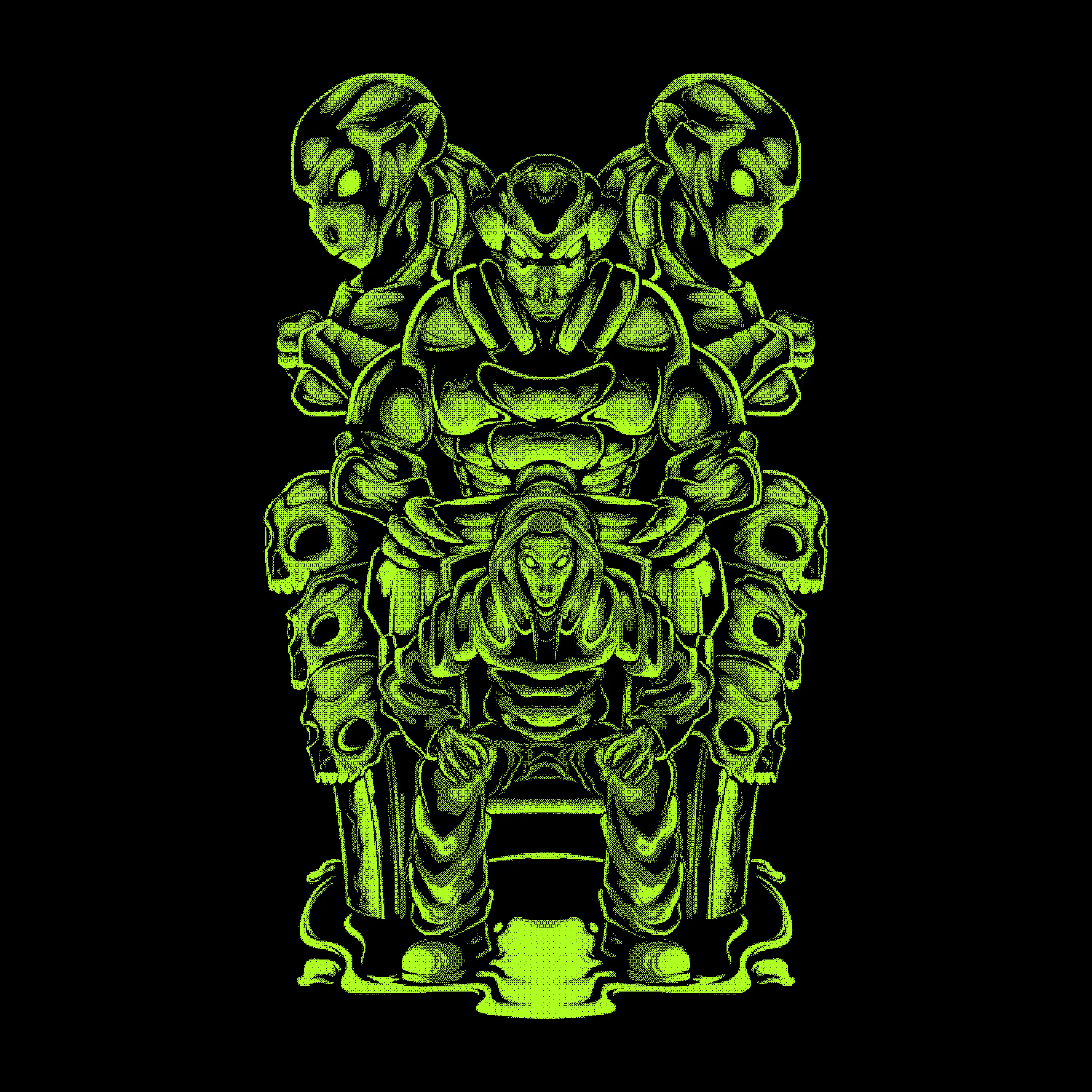
3. Cross-Site Request Forgery (CSRF)
Category: #Execution-Attack
Attack:
CSRF exploits a user's authenticated session to perform unauthorized actions without their knowledge.
Attack Code Example (CSRF Form Attack):
<form action="http://target-site.com/transfer" method="POST">
<input type="hidden" name="amount" value="10000">
<input type="hidden" name="to_account" value="attacker-account">
</form>
<script>
document.forms[0].submit();
</script>
When this malicious form is loaded in the victim's browser, it automatically submits a request to transfer funds to the attacker's account.
Vulnerable Code (No CSRF protection):
@app.route('/transfer', methods=['POST'])
def transfer():
# Process the transfer request without verifying the source
transfer_funds(request.form['to_account'], request.form['amount'])
Remediation Steps:
- CSRF Tokens: Use anti-CSRF tokens in all sensitive forms and validate them server-side.
- SameSite Cookies: Set cookies with the
SameSite
attribute to prevent them from being sent with cross-site requests. - Referer/Origin Header Validation: Validate the
Referer
orOrigin
headers to ensure requests are coming from trusted sources.
Safe Code (CSRF Token Validation in Python Flask):
@app.route('/transfer', methods=['POST'])
def transfer():
if not request.form['csrf_token'] == session['csrf_token']:
abort(403) # CSRF protection, reject the request if CSRF token doesn't match
# Process the transfer
transfer_funds(request.form['to_account'], request.form['amount'])
# Safe form with CSRF protection
@app.route('/form', methods=['GET', 'POST'])
def form():
csrf_token = generate_csrf_token()
session['csrf_token'] = csrf_token # Store CSRF token in session
return render_template('form.html', csrf_token=csrf_token)
Reference:
OWASP CSRF Prevention Cheat Sheet