| Tiago Coimbra
14. Buffer Overflow
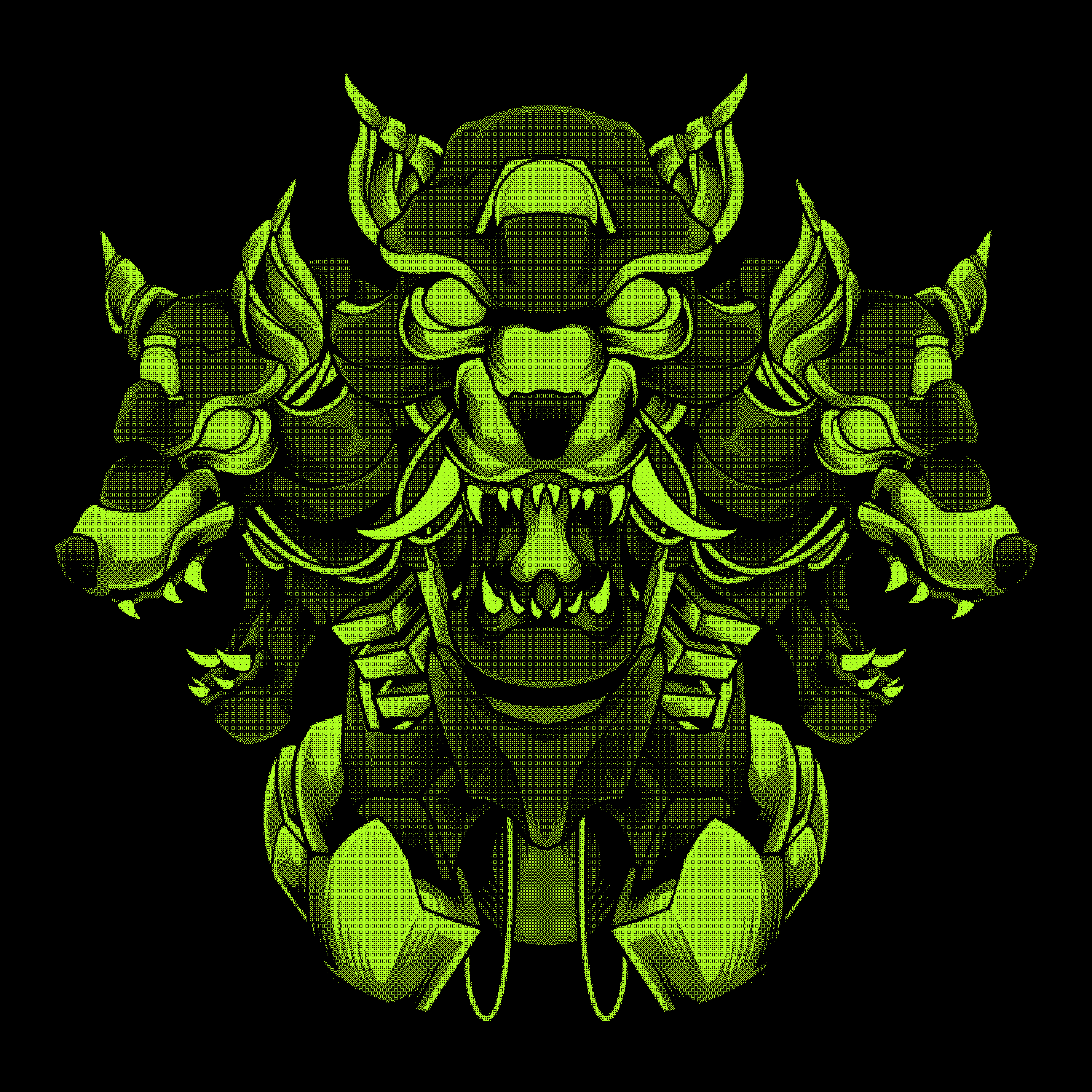
Buffer Overflow
Category: #Code-Execution-Attack
Attack: A buffer overflow occurs when data written to a buffer exceeds the buffer’s capacity, potentially allowing attackers to overwrite adjacent memory and execute arbitrary code or crash the application.
Attack Code Example (C-style Buffer Overflow):
#include <stdio.h>
#include <string.h>
int main() {
char buffer[8]; // Buffer with a fixed size of 8 bytes
strcpy(buffer, "This is a very long string that will overflow the buffer!");
printf("Buffer content: %s\n", buffer); // The overflow corrupts memory here
return 0;
}
In this C example, the string "This is a very long string that will overflow the buffer!"
exceeds the 8-byte buffer, leading to an overflow that may crash the program or allow arbitrary code execution.
Vulnerable Code Example:
void vulnerable_function(char *user_input) {
char buffer[16];
strcpy(buffer, user_input); // No bounds checking, allowing overflow
}
Remediation Steps:
- Use Safe Functions: Replace unsafe functions like
strcpy()
with safer alternatives such asstrncpy()
or use bounds-checking libraries. - Input Validation: Always validate the length of the input before writing it into a buffer.
- Use Modern Programming Languages: Use languages with automatic memory management (e.g., Python, Java) that are inherently safe from buffer overflow issues.
Safe Code Example (C with strncpy()
):
void safe_function(char *user_input) {
char buffer[16];
strncpy(buffer, user_input, sizeof(buffer) - 1); // Ensure no overflow
buffer[sizeof(buffer) - 1] = '\0'; // Null-terminate the string
}
Reference: OWASP Buffer Overflow Overview